Custom Events¶
The Custom event type Lets you create your own event using code.
For example, the OnTranslationEvent
class records the position of a target every time it moves.
public class OnTranslationEvent : CustomEventTypeDefinition
{
public GameObject Target { get; set; } = null;
private GameObject spawnedPrefab = null;
protected override void OnCreate()
{
Target = Resources.Load<GameObject>("TargetCube");
if (Target != null)
{
spawnedPrefab = AnalyticsUtilities.Spawn(Target);
spawnedPrefab.AttachCallback(AnalyticsUtilities.Hook.OnTranslation, (other, actions) =>
{
Data.RuntimePayload["PositionX"] = spawnedPrefab.transform.position.x.ToString();
Data.RuntimePayload["PositionY"] = spawnedPrefab.transform.position.y.ToString();
Data.RuntimePayload["PositionZ"] = spawnedPrefab.transform.position.z.ToString();
TriggerEvent();
});
}
}
}
Custom Event Configuration¶
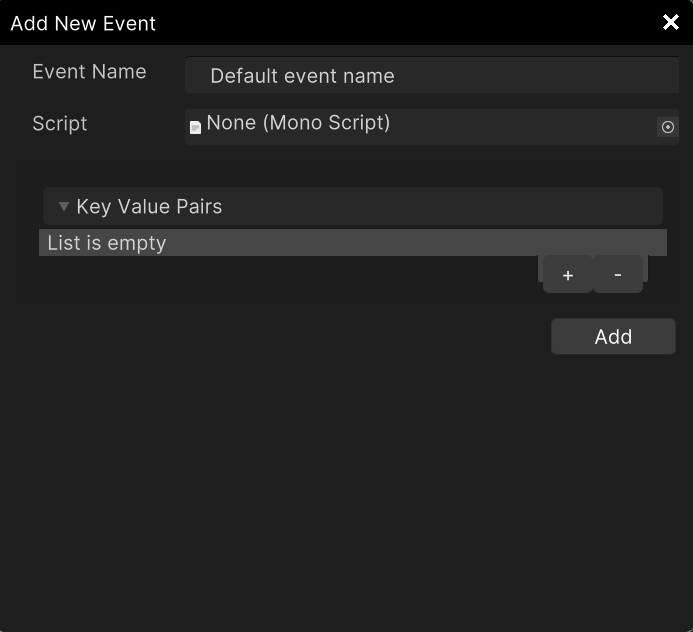
The Custom event contains the following configurable parameters:
Parameter Name |
Explanation |
---|---|
Event Name |
The name of the event. |
Script |
The script that implements the logic of the custom event. |