Errors¶
The Errors component allows developers to specify and evaluate distinct actions within the virtual environment, defining criteria for errors and their impact on the Action score.
Error Types¶
Time Limit:
Sets upper and lower time limits for an Action duration.
Triggers an error and applies a penalty to the Action score if limits are surpassed or not reached.
Collision:
Detects whether a specified prefab starts or stops colliding with a designated Collider.
Triggers an error and applies a penalty to the Action score.
Performed Wrong Action:
Triggers an error in case a specified target Action is performed while the selected Action is still in progress.
Applies a penalty to the Action score accordingly.
Did Not Perform Required Action:
Triggers an error if a specified target action is not executed while the selected Action is ongoing.
Applies a penalty to the Action score as defined by the developer.
Rotation Limit:
Monitors if a specified prefab exceeds the maximum allowed rotation.
Triggers an error and applies a penalty to the Action score.
Interaction:
Triggers an error if a specified interactable prefab is seized by a designated interactor prefab.
Applies a penalty to the Action score.
Range of Motion Limit:
Verifies if a specified prefab travels a distance exceeding the predefined limit.
Triggers an error and applies a penalty to the Action score.
Insertion Angle Error:
Exclusive to Insert Actions, activates if the insertion angle difference surpasses the specified limit.
Triggers an error and applies a penalty to the Action score.
Custom:
A Custom Error defined by implementing the CustomErrorTypeDefinition class and attaching it to the UI. For example, the HeightLimitError triggers if the Target prefab exceeds the specified MaxHeight.
public class HeightLimitError : CustomErrorTypeDefinition { public float MaxHeight { get; set; } = 2f; public GameObject Target { get; set; } = null; private GameObject spawnedPrefab = null; protected override void OnCreate() { Target = Resources.Load<GameObject>("TargetCube"); } protected override void OnActionInitialize(BaseActionData baseActionData) { if (baseActionData.ActionName == ActionName) { spawnedPrefab = AnalyticsUtilities.Spawn(Target); spawnedPrefab.AttachCallback(AnalyticsUtilities.Hook.OnTranslation, () => { if (spawnedPrefab.transform.position.y > MaxHeight) { TriggerError(); } }); } } protected override void OnActionPerform(BaseActionData baseActionData, bool skipped) { if (baseActionData.ActionName == ActionName) { AnalyticsUtilities.Destroy(spawnedPrefab); } } protected override void OnActionUndo(BaseActionData baseActionData) { if (baseActionData.ActionName == ActionName) { AnalyticsUtilities.Destroy(spawnedPrefab); } } }
How to Setup Errors¶
Time Limit, Collision, and Range of Motion Error¶
In this tutorial, we’ll guide you through configuring Time Limits, Collision Errors, and Range of Motion Errors for the Action “Pour Alcohol on cotton ball” in the MAGES Example Scene.
Navigate to the Analytics Editor Tab in the MAGES Panel.
Click on the Select Action dropdown and select the first Action.
Choose the Time Limit Error from the Error Type dropdown and click Add New Error.
Configure the error duration to 10 seconds and customize other settings as needed. Click Add to apply.
Your new Error will appear in the existing Errors field. Click on the
button to make adjustments.
Repeat the process for the Collision Error, paying attention to the Prefab and Collider fields.
Set the Cotton Ball prefab to prevent it from dropping on the floor.
Create a Floor Collider prefab and position it on the scene’s floor.
Set the Floor Collider prefab in the Collider Field.
For the Range of Motion Error, select the cotton ball in the target prefab field, set the Max allowed distance to 10, and add the Error.
Interaction and Rotation Error¶
This tutorial focuses on creating an Interaction and Rotation Error for the “activate syringe” Action in the MAGES Example Scene.
In the MAGES Panel navigate to
Analytics Editor > Errors > Select Action
, choose the “activate syringe” Action, then select the Interaction Error.Place the alcohol spray in the interactable field and assign the right hand prefab to the interactor. Replicate this process for the left hand by creating another Error.
With these configurations, any attempt to interact with the alcohol spray during the action will trigger an error.
To ensure the syringe maintains a consistent position on the patient’s arm throughout the activation phase, introduce the SyringeFinal prefab into the scene, representing the final position after the previous Action. Examine its current rotation.
In the Rotation Limit Error, set the syringe as the target prefab and apply the observed rotation limit from the syringe final prefab to maintain its stability.
Performed Wrong Action Error¶
This tutorial focuses on creating the “Performed Wrong Action” error for the “Pour Alcohol on the Cotton ball” Action. If the “R Cube 1” Action is performed while the “Pour Alcohol on the Cotton ball” Action is active, an error will be recorded.
In the MAGES Panel navigate to
Analytics Editor > Errors > Select Action
, select the “Pour Alcohol on the Cotton ball” Action and select the “Performed Wrong Action” Error.In the configuration tab, set the wrong Action to perform, specifying the Action related to moving the cube, which, in this case, is “R Cube 1.”
Adding this Error will record an error if the runtime Scenegraph ever reaches a state where the “R Cube 1” Action is performed while the “Pour Alcohol on the Cotton ball” Action is active.
Error Preview¶
After completing your operation, accessing the Session Overview UI provides a convenient means to review errors. Navigate to the UI spawned at the operation’s conclusion and click on the Errors tab to access a comprehensive list of Errors that occurred.
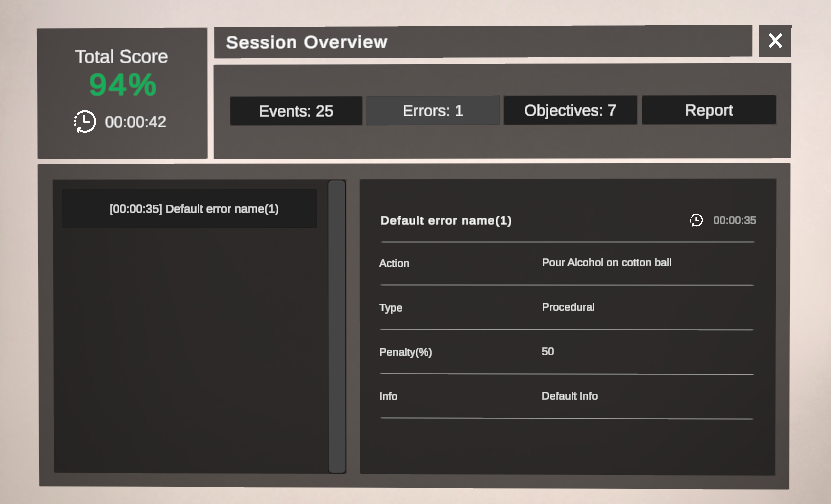