Events¶
The Events component lets you add extra details to your analytics using key-value pairs tied to specific Actions.
Event Types¶
On Action Perform
Records when a specific Action is performed.
On Action Undo
Records when a specific Action is undone.
On Collision
Records when a specified collision happens between a prefab and a collider. You can set it up to only record collisions under a specific Action and define the collision type.
On Interaction
Records when a specified interaction between an interactor and an interactable happens. You can set it up to record these interactions under a specific Action.
On Session Start
Records when the session starts.
On Session End
Records when the session ends.
Custom Event
Lets you create your own event using code. For example, the OnTranslationEvent class records the position of a target every time it moves.
public class OnTranslationEvent : CustomEventTypeDefinition { public GameObject Target { get; set; } = null; private GameObject spawnedPrefab = null; protected override void OnCreate() { Target = Resources.Load<GameObject>("TargetCube"); if (Target != null) { spawnedPrefab = AnalyticsUtilities.Spawn(Target); spawnedPrefab.AttachCallback(AnalyticsUtilities.Hook.OnTranslation, (other, actions) => { Data.RuntimePayload["PositionX"] = spawnedPrefab.transform.position.x.ToString(); Data.RuntimePayload["PositionY"] = spawnedPrefab.transform.position.y.ToString(); Data.RuntimePayload["PositionZ"] = spawnedPrefab.transform.position.z.ToString(); TriggerEvent(); }); } } }
How to Setup Events¶
For this tutorial, let’s set up 3 events to see how the pipeline works. We will create an On Action Perform, a Collision Event and a Session Ended event. Also, we will attach a custom recording to the on Action Perform event.
In the MAGES Panel, go to
Analytics Editor > Events tab
.Select Action Performed in the Event type dropdown, then click Add New Event. Name it, select the Action and click Add to apply.
Repeat for On Collision, setting the Collision type to enter and specifying the prefab and collider, then click Add to apply.
Repeat for Session Ended.
You can add custom key-value pairs to Events by creating a script that implements the
IEventDefinition
interface.public class GetHeartRate : IEventDefinition { /// <inheritdoc cref="IEventDefinition.Definition"/> public object Definition() { return 90; } }
Add this script to the list.
Event Preview¶
To view recorded Events, check the Session Overview UI generated at the operation’s conclusion. Click on the Events tab for a list of Events and their information.
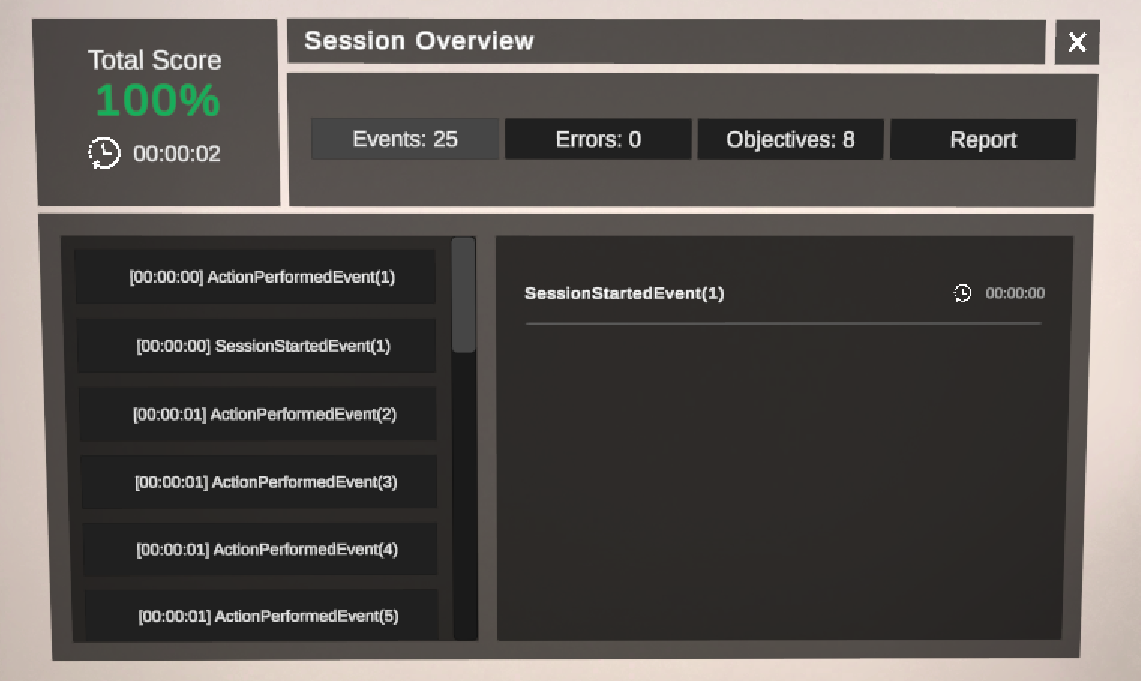